Unit 3 - Sound and Effects - Unity Learn
In this Unit, you will program a fast-paced endless side-scrolling runner game where the player needs to time jumps over oncoming obstacles to avoid crashing. In creating this prototype, you will learn how to add music and sound effects, completely transfo
learn.unity.com
Make player jump at start
플레이어에 힘과 중력을 적용하여 제어하려면 플레이어의 Rigidbody component의 메서드를 호출해야 한다.
void Start()
{
//initialize.
playerRb = GetComponent<Rigidbody>();
//use the AddForce method to make the player jump at the start of the game.
playerRb.AddForce(Vector3.up * 1000);
}
In the Player Rigid Body component, expand Constraints, then Freeze all but the Y position.
플레이어가 Y방향이 아닌 곳으로 움직이는 것을 방지
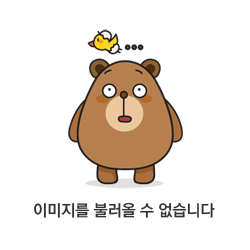
Fix background repeat with collider
BoxCollider를 Component로 추가하고 이를 통해 너비를 받아와 중간 지점을 찾는다.
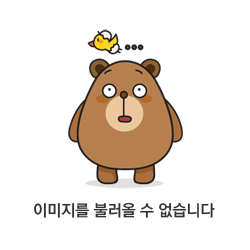
임의 값을 직접 입력하지 않고 Component의 Property로 기준을 설정한다.
void Start()
{
startPos = transform.position;
//BoxCollider를 가져와 너비 x의 중간값을 구하여 반복너비를 설정한다.
repeatWidth = GetComponent<BoxCollider>().size.x / 2;
}
Add a new game over trigger
Tag를 추가하여 충돌 Event가 발생하여 OnCollisionEnter가 호출될 때 충돌 객체를 구분한다
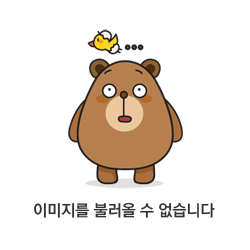
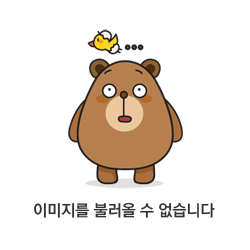
private void OnCollisionEnter(Collision collision)
{
if(collision.gameObject.CompareTag("Ground"))
{
isOnGround = true;
}
else if(collision.gameObject.CompareTag("Obstacle"))
{
Debug.Log("Game Over");
gameOver = true;
}
}
Stop MoveLeft on gameOver
장애물에 걸려 gameOver되면 Background 움직임이 멈춰야한다.
서로 다른 객체간의 커뮤니케이션 능력이 필요하다.
MoveLeft 클래스에 PlayerController 클래스 형태의 멤버를 선언한다.
GameObject의 멤버 메서드 Find에 “Player” 파라미터를 넘겨
Scene Hierarchy에 “Player”라는 이름의 객체를 찾고,
GetComponent 메서드로 받아와 멤버 변수를 초기화한다.
void Start()
{
playerControllerScript = GameObject.Find("Player").GetComponent<PlayerController>();
}
Set up a jump animation
점프 애니메이션을 Animator Component를 받아와 SetTrigger 메서드로 설정한다.
private Animator playerAnim;
void Start()
{
…
playerAnim = GetComponent<Animator>();
…
}
void Update()
{
if(Input.GetKeyDown(KeyCode.Space) && isOnGround)
{
…
playerAnim.SetTrigger("Jump_trig");
}
}
Adjust the jump animation
애니메이션을 좀 더 현실감을 주기 위해 Animator, 플레이어의 mass, jump force, gravity modifier를 조절한다.
점프애니메이션을 조정하기 위해 Animator에서 Running_Jump state의 스피드를 조절
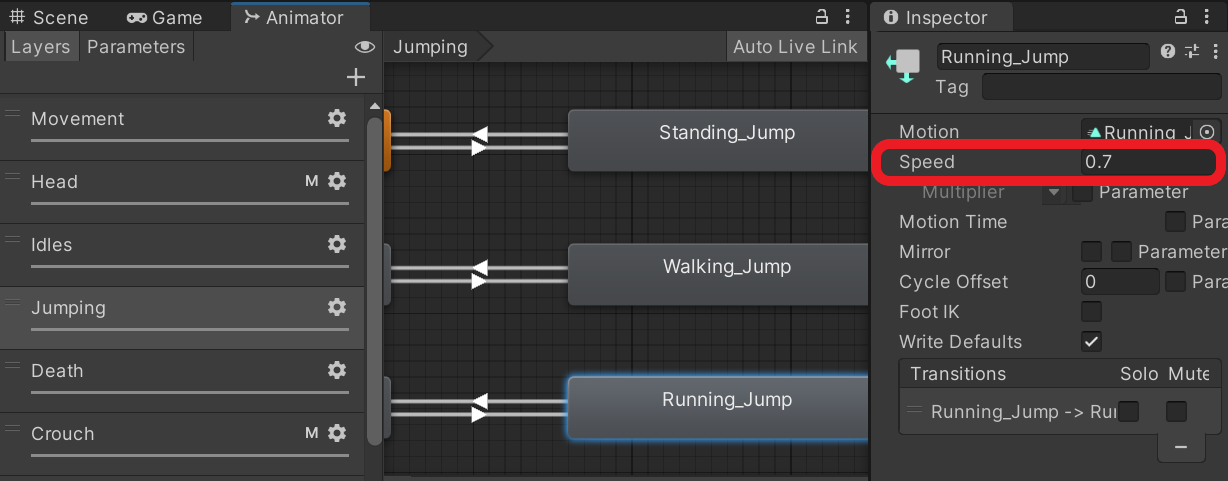
Rigidbody Mass 설정
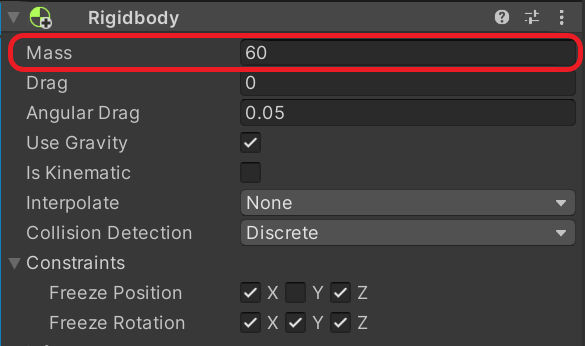
PlayerController 클래스 멤버변수 설정
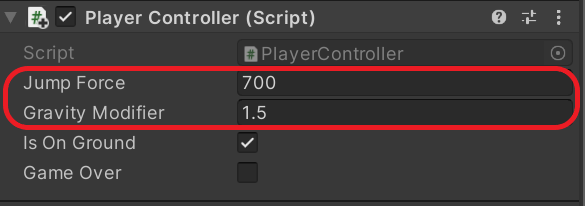
https://github.com/Myoungmin/UnityLearn-Create_with_Code-Prototype_3
GitHub - Myoungmin/UnityLearn-Create_with_Code-Prototype_3: Unit 3 - Sound and Effects
Unit 3 - Sound and Effects. Contribute to Myoungmin/UnityLearn-Create_with_Code-Prototype_3 development by creating an account on GitHub.
github.com
'Unity > Unity Learn' 카테고리의 다른 글
Junior Programmer > Unit 5 - User Interface (0) | 2022.06.18 |
---|---|
Junior Programmer > Unit 4 - Gameplay Mechanics (0) | 2022.06.15 |
Junior Programmer > Unit 2 - Basic Gameplay (0) | 2022.06.04 |
Junior Programmer > Unit 1 - Player Control (0) | 2022.06.01 |
Unity Essentials > Essentials of real-time audio (0) | 2022.05.31 |